Demystifying Blockchain Security: An Easy-to-Understand Guide
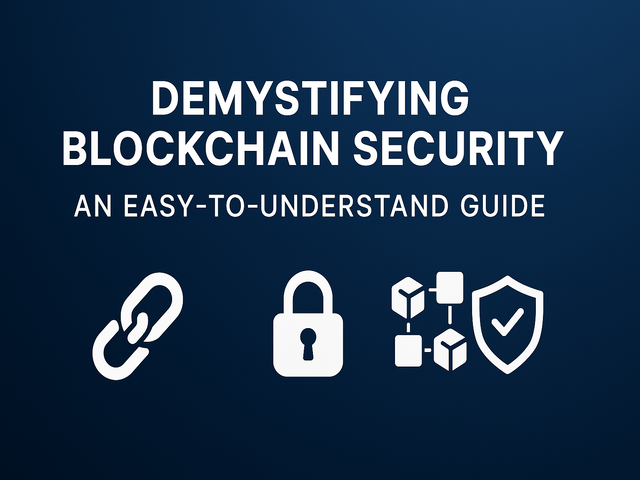
Blockchain is described as "unhackable" and "future-proof," but how is it that it's so secure? Let's break the magic of blockchain into plain language — with actual code examples — to give you a sense of what's happening under the hood.
Prefer watching instead of reading? Here’s a quick video guide
What Is a Blockchain?
A blockchain is a distributed digital ledger that stores transactions in a manner that makes them tamper-evident and irreversible.
Let's make a highly simplified version of a blockchain in Python to see how it works.
import hashlib
import time
class Block:
def __init__(self, index, data, previous_hash):
self.timestamp = time.time()
self.index = index
self.data = data
self.previous_hash = previous_hash
self.hash = self.calculate_hash()
def calculate_hash(self):
block_string = f"{self.index}{self.timestamp}{self.data}{self.previous_hash}"
return hashlib.sha256(block_string.encode()).hexdigest()
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
return Block(0, "Genesis Block", "0")
def add_block(self, data):
prev_block = self.chain[-1]
new_block = Block(len(self.chain), data, prev_block.hash)
self.chain.append(new_block)
# Create blockchain and add data
my_chain = Blockchain()
my_chain.add_block("Alice pays Bob 5 BTC")
my_chain.add_block("Bob pays Charlie 3 BTC")
# Print blockchain
for block in my_chain.chain:
print(vars(block))
Why Is Blockchain Secure?
- Immutability via Hashing: Every block has a cryptographic hash that identifies its data uniquely.
import hashlib
data = "Some transaction"
hash_val = hashlib.sha256(data.encode()).hexdigest()
print(hash_val)
When the data is altered even minutely, the hash value alters significantly — this makes tampering obvious.
- Chaining Blocks: Each block saves the hash of the last one. When a person attempts to alter one block, all the subsequent blocks are invalidated.
What Is a Consensus Mechanism?
Networks employ consensus algorithms to reach a consensus on what is added to the blockchain. Let's mimic Proof of Work, similar to Bitcoin.
class POWBlock(Block):
def mine_block(self, difficulty):
prefix = '0' * difficulty
while not self.hash.startswith(prefix):
self.timestamp = time.time()
self.hash = self.calculate_hash()
# Example usage
difficulty = 4
block = POWBlock(1, "Mining Example", "0")
block.mine_block(difficulty)
print(f"Mined hash: {block.hash}")
This mimics mining by compelling the hash to begin with a particular number of zeros — computational work is needed, which makes the network secure.
Typical Blockchain Security Risks (With Examples)
- Smart Contract Weaknesses: Smart contracts are computer code that executes automatically on blockchain platforms such as Ethereum. A straightforward buggy contract would appear as follows:
// Vulnerable Solidity Smart Contract
pragma solidity ^0.8.0;
contract SimpleBank {
mapping(address => uint) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw() public {
uint amount = balances[msg.sender];
payable(msg.sender).transfer(amount);
balances[msg.sender] = 0; // Should come before transfer
}
}
- Phishing Attacks: No code to display — just never share your private key or seed phrase, even if someone is trying to act like they're from MetaMask or a support group.
Identification of Tampering in Blockchain
def is_chain_valid(chain):
for i in range(1, len(chain)):
current = chain[i]
previous = chain[i-1]
if current.hash != current.calculate_hash():
return False
if current.previous_hash != previous.hash:
return False
return True
print("Is blockchain valid?", is_chain_valid(my_chain.chain))
# Try tampering
my_chain.chain[1].data = "Alice pays Eve 100 BTC"
print("Is blockchain valid after tampering?", is_chain_valid(my_chain.chain))
Security Tips for Blockchain Users
- Use Hardware Wallets: Keeps your private keys offline.
- Enable 2FA on Exchanges: Adds a second layer of protection.
- Avoid Suspicious Links: Bookmark official DApps and wallets.
- Double-check Smart Contracts: Before using DeFi platforms.
How Real Projects Improve Security
- Code Audits: Professional firms analyze smart contracts.
- Bug Bounties: Ethical hackers disclose vulnerabilities.
- Multi-sig Wallets: Need multiple signatures for fund transfer.
- Security Libraries: Employ tried and tested cryptographic libraries and tools.
Following is an example of a multi-signature wallet logic in pseudocode:
class MultiSigWallet:
def __init__(self, owners, required_signatures):
self.owners = set(owners)
self.required = required_signatures
self.transactions = []
def submit_transaction(self, tx, signatures):
if len(set(signatures) & self.owners) >= self.required:
self.transactions.append(tx)
print("Transaction approved and recorded.")
else:
print("Not enough valid signatures.")
Conclusion: Is Blockchain Unhackable?
No. But it's among the most secure tech out there — when used and done right. The greatest threats tend to be outside the blockchain itself, including:
- Defective smart contract reasoning
- User error
- Insecure wallets
- Weak governance
Blockchain foundations (decentralization + cryptography) are sound. Your job is to remain attentive, learn about risks, and use reputable tools.
Summary
- Hashing: Creates a unique digital fingerprint for every block
- Chaining: Connects blocks with the help of the preceding hash
- Proof of Work: Demands computational power to authenticate blocks
- Smart Contracts: Automated programs that require auditing
- Tampering Detection: Change detection through hash confirmation
- User Safety: Secure wallets, phishing protection, 2FA
Want to Learn More?
- Try coding a mini blockchain in Python.
- Master Solidity and deploy contracts to testnets.
- Track security-oriented blockchain platforms (such as Trail of Bits, OpenZeppelin).
- Participate in bug bounty platforms (such as Immunefi) to help make blockchain more secure.